Never lose track of your deployed versions again !
Nowadays, with the entire containerized ecosystem, we often have black boxes exposing our applications. Our applications are packaged in Docker images, making it challenging for developers, QA testers, clients, or anyone on the team to easily see what’s inside. And you may also have continuous deployment that will deploy automatically the code. Add to this rollback strategies, and so on.
With all these mechanisms in place, have you ever wondered,
Am I sure that my .js is the expected version?
On which version was the user test performed?
Here are some tips to display the version of your application (and make everyday work easier for your entire team), in a more or less hidden way.
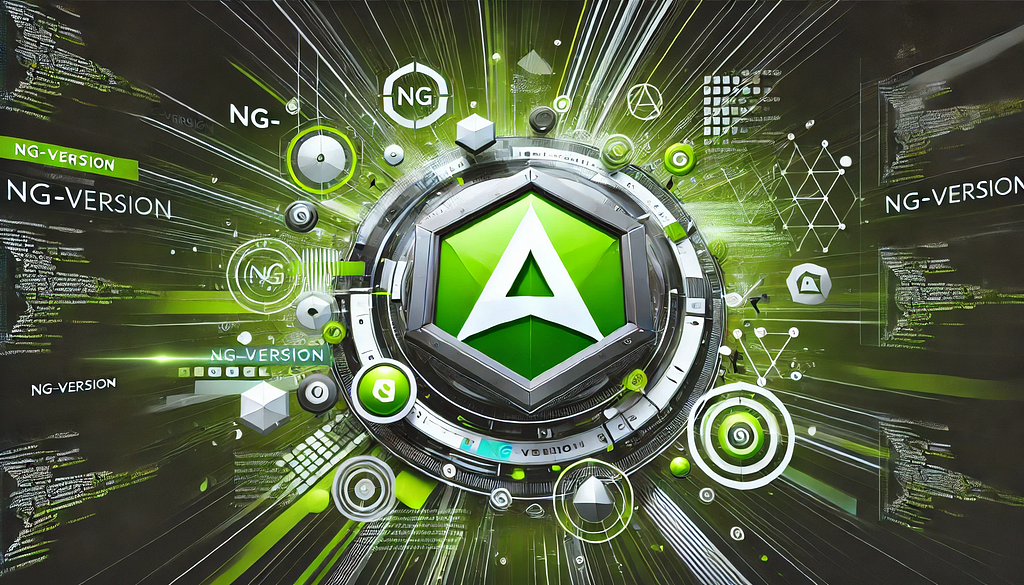
Add the version on the application’s root element
One way could be to expose the version as Angular does, in a DOM attribute of your root element by retrieving the version from your package.json.
The first step will be to be able to access the version written in the package.json. To ensure the .json file is resolved by the TypeScript compiler, we add this configuration in our tsconfig.app.json file.
"compilerOptions": {
"resolveJsonModule": true
}
If you don’t, you may face this Typescript error.
Cannot find module '../../../../package.json'.
Consider using '--resolveJsonModule' to import module with '.json' extension.
And since we might want to (why not?) use the content of our package.json in different parts of our application (and because I like aliases for imports), let’s add an alias to our package.json!
"paths": {
"packageJson": ["./package.json"]
}
- Then in our app.component.ts, we simply need to import the version property we are interested in.
import { version } from "packageJson";
- To display it in our DOM Node, let’s use Angular’s API with the decorator @HostBinding
@HostBinding('attr.app-version') appVersion = version;
Now, start our application to see the result.
npm start
Et voilà !

Display on the page
Using the same mechanism, if we have an application with its own page, we can imagine displaying the application’s version in the footer.
- Set a public property in our Angular @Component
appVersion = version;
- Use the interpolation to display the value in the template.
<main>
<h1>Welcome in our testing application !</h1>
</main>
<footer>Current version: {{ appVersion }}</footer>

Fetch the version from the source manager (in this example, git)
It may happen that the version of our application is not carried by the package.json, but by git (often a tag). In this case, retrieving it via the package.json is not feasible.
To simplify, we will use the @ngx-env/builder library to easily access environment variables.
First step, add the library to our dependencies.
ng add @ngx-env/builder
This step will automatically create a typing file in your “src/”.

It will help you just after to strictly type what you are expecting in the environment variables.
The file itself is well documented, just add and remove what you want, here is what it looks like in our example.
// Define the type of the environment variables.
declare interface Env {
readonly NODE_ENV: string;
// Replace the following with your own environment variables.
// Example: NGX_VERSION: string;
NG_APP_TAG_VERSION: string;
}
// Choose how to access the environment variables.
// Remove the unused options.
// 1. Use import.meta.env.YOUR_ENV_VAR in your code. (conventional)
declare interface ImportMeta {
readonly env: Env;
}
Then create a git tag in our repository.
git tag v2.0.0-article
Finally, in our main component, retrieve the environment variable.
tagVersion = import.meta.env["NG_APP_TAG_VERSION"];
And expose it in the template.
<footer>Current version: {{ tagVersion }}</footer>
All that’s left is to adapt your pipeline to retrieve the tag name, depending on the infrastructure you’re using, you may have some variables at your disposal. Otherwise, you can retrieve the information on your own using this command.
git describe --exact-match --tags
So when building your application, you can just adapt your ng build as follows!
NG_APP_TAG_VERSION=`git describe --exact-match --tags` npm run build

Bonus
If we want to use it in a Docker’s file:
FROM node:<node_version or latest> as build
ARG NG_APP_TAG_VERSION
WORKDIR build
COPY . .
RUN npm ci
RUN npm run build
FROM nginx:alpine as final
COPY --from=build build/dist/article-appversion/browser /usr/share/nginx/html
EXPOSE 80
ENTRYPOINT ["nginx", "-g", "daemon off;"]
And during the creation of the image, provide the environment variable.
docker build -t article-version . --build-arg NG_APP_TAG_VERSION=$=`git describe --exact-match --tags`
Conclusion
We might want to display different metadata in our application. This article is an introduction to the possibilities available to us, feel free to share yours!
Versions used
Angular : 18.1.3
Typescript : 5.5.2
Node : 20.11.0
Package Manager : npm 10.2.4
For more updates, visit ekino’s website and follow us on LinkedIn.
Display the version of a deployed Angular application was originally published in ekino-france on Medium, where people are continuing the conversation by highlighting and responding to this story.